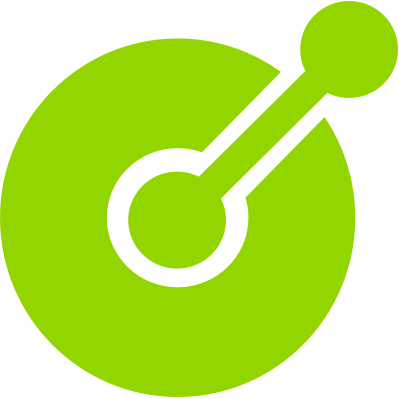
openapi-backend
Build, Validate, Route, Authenticate, and Mock using OpenAPI definitions.
OpenAPI Backend is a Framework-agnostic middleware tool for building beautiful APIs with OpenAPI Specification.
Features
- Build APIs by describing them in OpenAPI specification
- Register handlers for operationIds to route requests in your favourite Node.js backend
- Use JSON Schema to validate API requests and/or responses. OpenAPI Backend uses the AJV library under the hood for performant validation
- Register Auth / Security Handlers for OpenAPI Security Schemes to authorize API requests
- Auto-mock API responses using OpenAPI examples objects or JSON Schema definitions
- Built with TypeScript, types included
- Optimised runtime routing and validation. No generated code!
- OpenAPI 3.1 support
Quick Start
The easiest way to get started with OpenAPI Backend is to check out one of the examples.
npm install --save openapi-backend
import OpenAPIBackend from "openapi-backend";
// create api with your definition file or object
const api = new OpenAPIBackend({ definition: "./petstore.yml" });
// register your framework specific request handlers here
api.register({
getPets: (c, req, res) => res.status(200).json({ result: "ok" }),
getPetById: (c, req, res) => res.status(200).json({ result: "ok" }),
notFound: (c, req, res) => res.status(404).json({ err: "not found" }),
validationFail: (c, req, res) =>
res.status(400).json({ err: c.validation.errors }),
});
// initalize the backend
api.init();